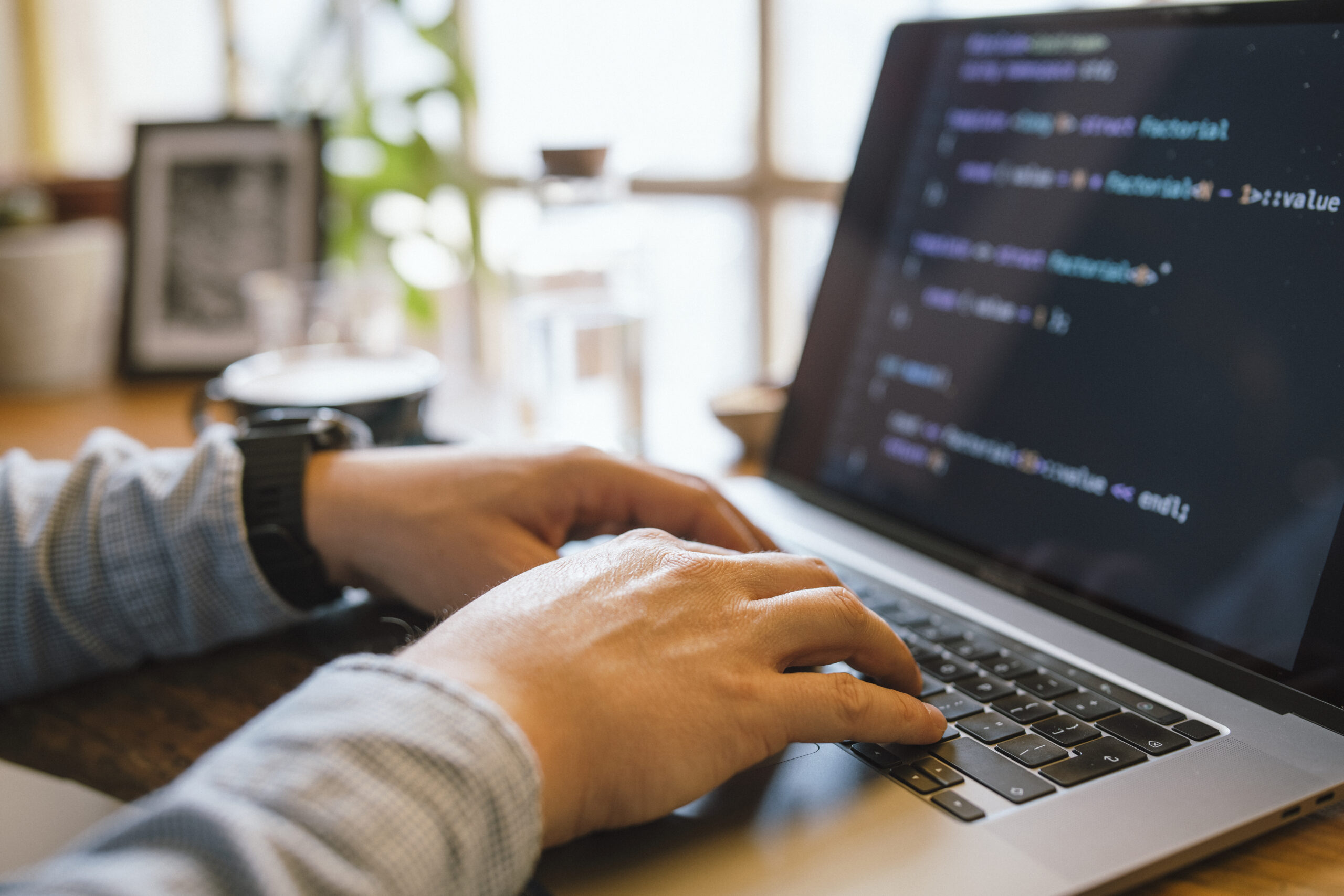
Debugging is Probably the most vital — nonetheless frequently disregarded — capabilities in a very developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Finding out to Assume methodically to resolve problems proficiently. No matter whether you are a starter or simply a seasoned developer, sharpening your debugging skills can conserve hours of stress and radically improve your productivity. Listed here are numerous tactics that can help builders stage up their debugging video game by me, Gustavo Woltmann.
Grasp Your Instruments
Among the quickest approaches builders can elevate their debugging capabilities is by mastering the resources they use every single day. Even though composing code is just one Section of advancement, realizing how you can communicate with it efficiently throughout execution is Similarly significant. Present day advancement environments come Geared up with strong debugging capabilities — but many builders only scratch the surface area of what these applications can perform.
Get, as an example, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code around the fly. When made use of appropriately, they Permit you to observe specifically how your code behaves all through execution, that's a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, check out serious-time functionality metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip discouraging UI issues into manageable jobs.
For backend or procedure-level developers, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Regulate over working procedures and memory administration. Learning these resources could possibly have a steeper learning curve but pays off when debugging efficiency troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become comfy with Edition Management units like Git to understand code historical past, come across the precise instant bugs were being introduced, and isolate problematic modifications.
In the end, mastering your applications means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement setting making sure that when difficulties crop up, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out fixing the particular challenge in lieu of fumbling by the procedure.
Reproduce the situation
One of the most critical — and infrequently forgotten — techniques in productive debugging is reproducing the situation. Ahead of jumping into the code or making guesses, builders need to have to make a constant ecosystem or state of affairs the place the bug reliably appears. Without the need of reproducibility, correcting a bug turns into a sport of chance, generally resulting in wasted time and fragile code changes.
Step one in reproducing an issue is accumulating just as much context as you possibly can. Talk to inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve collected enough data, attempt to recreate the situation in your local natural environment. This might necessarily mean inputting the identical data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the sting circumstances or point out transitions involved. These exams don't just assist expose the situation but also avoid regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd happen only on specific running systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more proficiently, take a look at probable fixes properly, and converse extra Evidently together with your group or customers. It turns an abstract complaint right into a concrete obstacle — Which’s where by builders prosper.
Read through and Recognize the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Incorrect. Instead of seeing them as disheartening interruptions, builders need to understand to treat mistake messages as immediate communications through the technique. They usually tell you what precisely transpired, wherever it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the information thoroughly and in full. Lots of developers, especially when underneath time strain, glance at the main line and promptly start off generating assumptions. But deeper during the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and fully grasp them initial.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These concerns can tutorial your investigation and stage you towards the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some glitches are vague or generic, As well as in Those people instances, it’s critical to look at the context by which the error happened. Check connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Properly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, encouraging you comprehend what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Popular logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard functions (like profitable commence-ups), WARN for potential challenges that don’t split the appliance, ERROR for precise troubles, and Deadly when the procedure can’t continue on.
Stay away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your system. Deal with essential occasions, point out alterations, input/output values, and critical conclusion factors in your code.
Structure your log messages clearly and continuously. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t feasible.
In addition, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Which has a properly-assumed-out logging strategy, you could decrease the time it's going to take to spot troubles, attain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not simply a technological job—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to approach the process like a detective fixing a thriller. This way of thinking assists break down sophisticated difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect as much relevant info as you are able to with out jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s occurring.
Following, variety hypotheses. Check with on your own: What may very well be resulting in this habits? Have any adjustments not too long ago been produced for the codebase? Has this concern occurred before less than very similar conditions? The aim is always to narrow down alternatives and establish likely culprits.
Then, examination your theories systematically. Make an effort to recreate the issue inside of a managed surroundings. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and Allow the effects direct you closer to the reality.
Spend shut focus to small facts. Bugs usually disguise while in the least predicted places—just like a missing semicolon, an off-by-a person error, or simply a race issue. Be thorough and individual, resisting the urge to patch the issue with no fully knowledge it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Lastly, preserve notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and assist Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering concealed challenges in complicated programs.
Produce Tests
Creating exams is among the simplest ways to boost your debugging capabilities and In general improvement efficiency. Exams not merely enable capture bugs early but will also function a security Web that gives you self-confidence when producing alterations to your codebase. A nicely-tested application is easier to debug since it permits you to pinpoint specifically the place and when a difficulty happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know the place to search, substantially decreasing the time used debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand staying mounted.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be sure that a variety of elements of your software operate with each other smoothly. They’re significantly valuable for catching bugs that happen in complex devices with several factors or companies interacting. If one thing breaks, your checks can inform you which part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to think critically about your code. To check a attribute properly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of comprehension naturally qualified prospects to raised code framework and much less bugs.
When debugging a problem, producing a failing check that reproduces the bug is often a powerful initial step. When the test fails persistently, you can target correcting the bug and view your examination go when the issue is settled. This tactic makes certain that the identical bug doesn’t return Sooner or later.
To put it briefly, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, striving Option after solution. But Just about the most underrated debugging applications is solely stepping absent. Having breaks helps you reset your mind, decrease aggravation, and often see The problem from a new viewpoint.
When you are also near to the code for also extended, cognitive tiredness sets in. You could commence overlooking clear mistakes or misreading code which you wrote just hours earlier. In this point out, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso split, and even switching to a special job for ten–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, In particular for the duration of lengthier debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping absent enables you to return with renewed Electrical power and also a clearer attitude. You might quickly recognize a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver about, extend, or do something unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, however it in fact leads to speedier and more effective debugging Eventually.
To put it briefly, using breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is a component of fixing it.
Master From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing worthwhile for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring yourself a couple of crucial queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code reviews, or logging? The responses often expose blind places inside your workflow or knowing and allow you to Create more robust coding behavior relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see patterns—recurring challenges or prevalent faults—which you could proactively keep away from.
In group environments, sharing what you've learned from the bug using your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, assisting others steer clear of the identical issue boosts staff effectiveness and cultivates a much better Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who produce excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging skills will take time, exercise, and patience — nevertheless read more the payoff is large. It makes you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.